The Software
I wrote some simple Python code to help with designing the velomobile. It takes a series of cross sections and outputs the shape of the panels that must be made to form the shape. It can be downloaded here (right-click, save as, change extension to .py): velo.html.
Input File
The input format is a file in which each line is: a b1 c1 b2 c2 b3 c3... where "a" is the position of a given cross section along the length of the velo and each (bN, cN) pair represents a corner of the cross section.
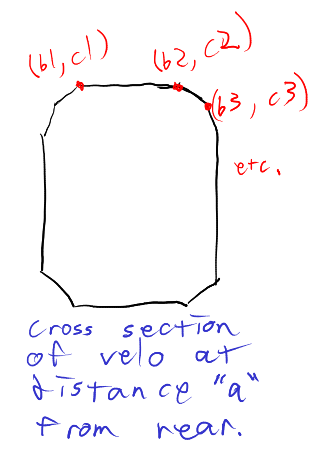
This is a finickiy input format - all the cross sections have to be written out as if they were on the same coordinate system. It isn't pretty. It's easy to make mistakes. But it works. My velo is represented by:
250 | -2 40 | 2 40 | 4 39 | 5 37.5 | 5 21 | 3 20 | -3 20 |
185 | -18 45 | 18 45 | 22 43 | 26 37 | 26 0 | 16 -10 | -16 -10 |
135 | -20 45 | 20 45 | 32 39 | 38 30 | 38 -2 | 20 -20 | -20 -20 |
65 | -20 40 | 20 40 | 2 34 | 38 25 | 38 -2 | 20 -20 | -20 -20 |
20 | -12 28 | 12 28 | 16 26 | 20 20 | 20 -2 | 12 -10 | -12 -10 |
0 | 0 0 | 0 0 | 0 0 | 0 0 | 0 0 | 0 0 | 0 0 |
Note the row of zeros at the end - that's where all the panels converge to a single point at the front. For some reason it doesn't like to have this as the first line, so I had to invert everything (this is probably fixable, but I didn't have any reason to). Also note that none of these cross sections is complete: The velo is symmetrical, so I only input one side. As an example, the first line, when drawn out, would look something like...
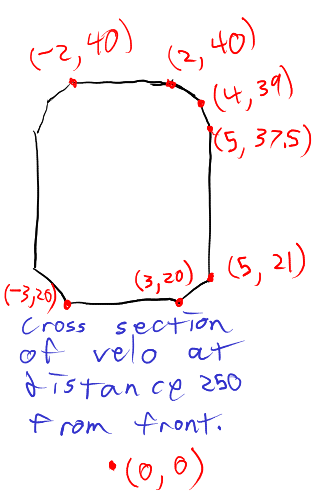
If you noticed that "rear" changed to "front" in the text under the drawing, congratulations! The first image is correct, since "0" in this example is the front of the velomobile. I'm just too lazy to fix this one.
Running the Program
If there's any interest in this I can give it a more sophisticated UI, but right now it's a simple Python script. (If don't have Python, you can install it from http://wiki.python.org/moin/BeginnersGuide/Download.) Open up a terminal (probably "Command Prompt" on Windows; Mac users should find Terminal in Applications/Utilites/) and run "python velo.py input_file_name".
If you want to build a scale model, you can edit line 64:
print str(round(bulkhead[0][0], 1)), '|', str(round(bulkhead[0][1], 1)) + ',', round(bulkhead[1][1], 1)
to read:
print str(round(bulkhead[0][0], 1)/XXX), '|', str(round(bulkhead[0][1], 1)/XXX) + ',', round(bulkhead[1][1], 1)/XXX
where XXX is the scale factor. For my scale model I used "/4". Make sure you replace all three instances of XXX!
Output
The result looks something like:
kg6gfq@asimov:~/Desktop/bike$ python velo.py velometric 0: 0.0 | 18.0, 22.0 65.2 | 2.0, 38.0 115.2 | 0.0, 40.0 185.4 | 0.0, 40.0 231.9 | 8.0, 32.0 266.4 | 20.0, 20.0 1: 0.0 | 0.0, 2.2 66.0 | 12.1, 16.5 116.0 | 13.9, 27.3 186.2 | 16.1, 29.5 233.4 | 14.3, 18.8 269.8 | 16.1, 16.1 2: 0.0 | 0.0, 1.8 67.2 | 6.7, 13.9 117.6 | 15.5, 26.3 187.7 | 19.7, 30.5 236.0 | 17.5, 24.7 270.2 | 30.2, 30.2 3: 0.0 | 0.0, 16.5 68.3 | 0.5, 37.5 119.7 | 7.5, 39.5 189.7 | 12.5, 39.5 238.2 | 17.5, 39.5 266.5 | 37.5, 37.5 4: 0.0 | 33.3, 35.5 70.8 | 23.9, 37.3 120.1 | 8.9, 35.2 189.5 | 0.0, 24.1 237.7 | 5.4, 17.1 264.5 | 14.6, 14.6 5: 0.0 | 17.0, 23.0 71.6 | 4.0, 36.0 122.6 | 0.0, 40.0 192.6 | 0.0, 40.0 238.7 | 8.0, 32.0 261.0 | 20.0, 20.0
Each numbered section represents one side (I think "chine" is the boatbuilding term) of the shell. Returning to the example cross section:
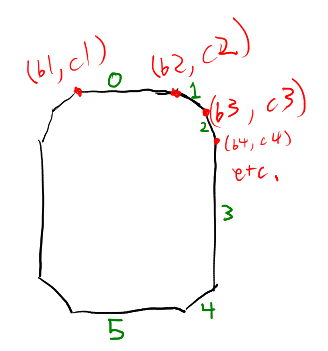
Each line in the section is a set of coordinates - so "0.0 | 18.0, 22.0
" should be read as "plot points (0, 18.0) and (0, 22.0)". Actually plotting all of these onto sheets of plywood in a space-efficient manner is a pain, and involves lots of shifting (adding/subtracting) and, if you're dealing with a design that crosses two sheets of plywood, as I am, also a lot of interpolation to figure out where the line is when it hits the edge of a sheet. It's just loads of fun...
If you want, you can comment out line 64 (that is, put a "#" in front of it) and uncomment line 65 (remove the "#") to get a CSV file. As far as I can tell this is only useful if you want to do spreadsheet operations on the result, or maybe if you want to graph the shape of the result (which could be handy for printing an enlarged version and tracing it onto the wood).